Digital Tachometer using IR Sensor with Arduino for measuring RPM
In this project, we have designed Digital Tachometer using IR Sensor with Arduino for measuring the number of rotations of rotating Motor in RPM. Simply we have interfaced IR sensor module with Arduino and SSD 1306 LCD module for display. The IR sensor module consists of IR Transmitter & Receiver in a single pair that can work a Digital Tachometer for speed measurement of any rotating object.
Components:
1) Arduino Nano: https://roboman.in/59h4
2) Arduino Nano Type C : https://roboman.in/xkfu
3) TP-4056 Module : https://roboman.in/oa0m
4) DC-DC Boost Converter LM2587 or XL6019 : https://roboman.in/kkuj
5) MAX7219 Dot Matrix 4 in 1 Display Module: https://roboman.in/jekd
6) Sound Sensor : Sound Sensor :https://roboman.in/vs1e
7) 18650 Lithium Battery 2500mah 3C :https://roboman.in/utwc
7) 18650 Lithium Battery 2600mah 3C :https://roboman.in/mmab
7) 18650 Lithium Battery 2200mah 1C :https://roboman.in/70b5
7) 18650 Lithium Battery 2000mah 1C :https://roboman.in/7em9
7) 18650 Lithium Battery 1800mah 1C :https://roboman.in/ywss
Diagram :
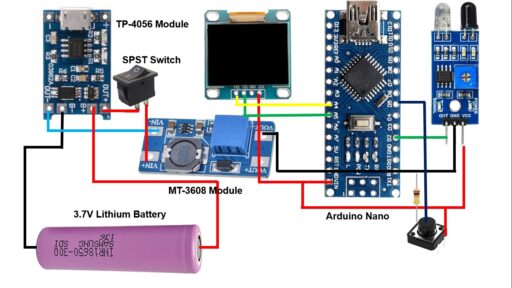
Code: